在我们的编程系列的延续中,我们将讨论函数(Function)——它所包含的大部分内容。如果您想学习如何编码,那么了解函数非常重要。这同样适用于那些倾向于经常复制他们的代码以用于他们工作的不同部分的当前程序员。
学习如何使用函数意味着编码人员将知道如何更有效地工作。不仅如此,代码将更易于阅读,如果您在团队中工作,这是一个福音。
什么是编程中的函数?
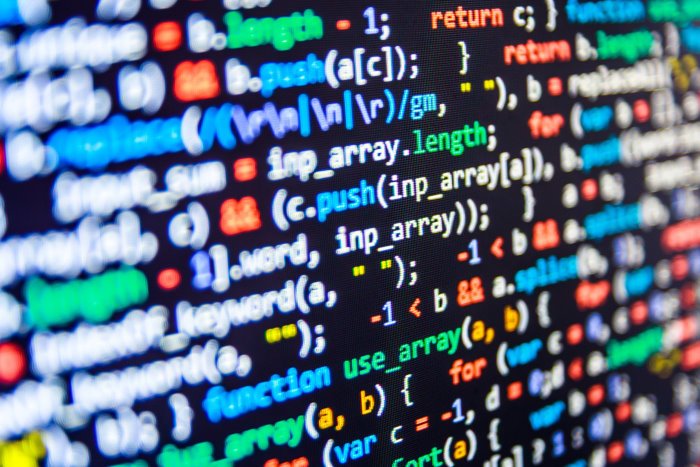
在基本术语中,函数是执行各种任务的代码块。如果需要,可以多次调用和重用一个函数。为了让事情变得更有趣,编码人员可以轻松地将信息传递给函数,但不仅如此,还可以直接返回信息。
目前,许多流行的编程语言都内置了此功能,这是预期的。
现在,每当调用一个函数时,程序通常会暂停当前正在运行的程序并执行该函数。从那里,函数将从上到下读取,一旦函数完成其任务,程序将从暂停的地方继续。
如果函数发回一个值,那么该特定值将在最初调用该函数的地方使用。
阅读(Read):什么是 Java 编程语言(What is Java Programming language)?
如何编写一个空函数
好的,所以编写一个 void 函数非常容易,并且可以在很短的时间内完成。请记住(Bear),此函数不返回值。让我们看一些示例,这些示例可能会让您知道该怎么做。
JavaScript 示例
function helloFunction(){
alert("Hello World!");
}
helloFunction();
Python 示例
def helloFunction():
print("Hello World")
helloFunction()
C++ 示例
#include <iostream>
using namespace std;
void helloFunction(){
cout << "Hello World!";
}
int main(){
helloFunction();
return 0;
}
阅读(Read): 什么是 R 编程语言(What is the R programming language)?
如何编写需要值的函数
如果您在整个工作中多次编写同一段代码,那么 void 函数非常适合。然而,这些类型的函数并没有改变,这并没有让它们变得超级有用。使 void 函数更有益的最好方法是通过向函数发送不同的值来增加它们可以做的事情。
Python 示例
def helloFunction(newPhrase):
print(newPhrase)
helloFunction("Our new phrase")
JavaScript 示例
function helloFunction(newPhrase){
alert(newPhrase);
}
helloFunction("Our new phrase");
C++ 示例
#include <iostream>
using namespace std;
void helloFunction(string newPhrase){
cout << newPhrase;
}
int main(){
helloFunction("Our new Phrase");
return 0;
}
阅读(Read):所有程序员都应该遵循的最佳编程原则和指南(Best Programming Principles & Guidelines all Programmers should follow)。
如何编写一个返回值的函数
那么,本文的最后一个方面是如何编写一个返回值的函数。每当您想在使用之前更改数据时,这就是大多数情况下的方法。
Python 示例
def addingFunction(a, b):
return a + b
print(addingFunction(2, 4))
JavaScript 示例
function addingFunction(a, b){
return a + b;
}
alert(addingFunction(2, 4));
C++ 示例
#include <iostream>
using namespace std;
int addingFunction(int a, int b){
return a + b;
}
int main(){
cout << addingFunction(2, 4) ;
return 0;
}
阅读(Read):初学者程序员的最佳项目(The best projects for beginner Programmers)。
玩得(Have)开心测试我们在此处列出的代码。我们希望它们对您的工作有用。
What is a Function in Programming? We explain
In continuation with our programming series, we are going to talk about Function – much of all that it entails. If you want to learn how to code, then understanding functions is really important. The same applies to current programmers who tend to copy their code quite often to use in a different section of their work.
Learning how to use functions means the coder will know how to work more efficiently. Not only that, but the code will be easier to read, and that is a boon if you’re working in a team.
What is a Function in Programming?
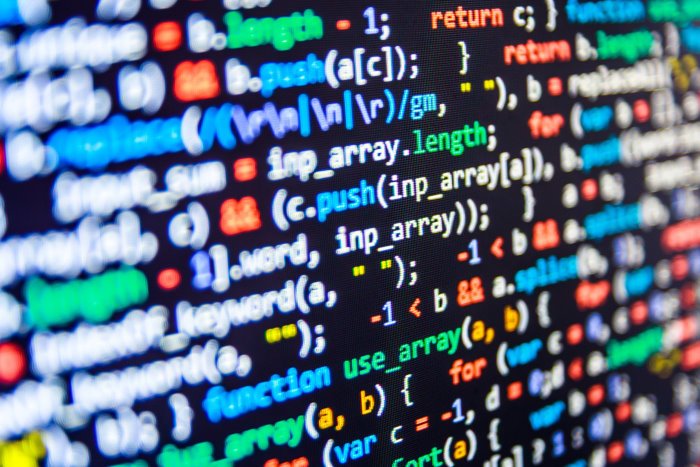
In basic terms, a function is a block of code that performs various tasks. Should you need to, a function can be called and reused numerous times. To make things even more interesting, coders can pass information to a function with ease, but not only that, but it is also possible to send information right back.
At the moment, many of the popular programming languages have this feature built-in, which is expected at this point.
Now, whenever a function is called, the program will usually pause the currently running program and implement the function. From there, the function will be read from top to bottom, and once the function has completed its task, the program will continue from where it had paused.
Should the function send back a value, that particular value will then be used where the function was originally called.
Read: What is Java Programming language?
How to write a Void function
OK, so writing a void function is super easy and can be done in a short amount of time. Bear in mind that this function does not return a value. Let’s look at a few examples that might give you an idea of what to do.
JavaScript Example
function helloFunction(){
alert("Hello World!");
}
helloFunction();
Python Example
def helloFunction():
print("Hello World")
helloFunction()
C++ Example
#include <iostream>
using namespace std;
void helloFunction(){
cout << "Hello World!";
}
int main(){
helloFunction();
return 0;
}
Read: What is the R programming language?
How to write Functions that require a value
If you are writing the same piece of code several times throughout your work, then void functions are perfect for that. However, these types of functions do not change, which doesn’t make them super useful. The best way to make void functions more beneficial is to increase what they can do by sending different values to the function.
Python Example
def helloFunction(newPhrase):
print(newPhrase)
helloFunction("Our new phrase")
JavaScript Example
function helloFunction(newPhrase){
alert(newPhrase);
}
helloFunction("Our new phrase");
C++ Example
#include <iostream>
using namespace std;
void helloFunction(string newPhrase){
cout << newPhrase;
}
int main(){
helloFunction("Our new Phrase");
return 0;
}
Read: Best Programming Principles & Guidelines all Programmers should follow.
How to write a Function that returns a value
The final aspect of this article, then, is how to write a function that will return a value. Whenever you want to alter data before using it, then this is the way to go in most situations.
Python Example
def addingFunction(a, b):
return a + b
print(addingFunction(2, 4))
JavaScript Example
function addingFunction(a, b){
return a + b;
}
alert(addingFunction(2, 4));
C++ Example
#include <iostream>
using namespace std;
int addingFunction(int a, int b){
return a + b;
}
int main(){
cout << addingFunction(2, 4) ;
return 0;
}
Read: The best projects for beginner Programmers.
Have fun testing the codes we’ve listed here. We hope they will prove useful in your work.